|
 |
Delphi programming while do loop to search array |
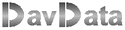 |
 |
This article presents a simple function that searches for specific data in an array.
As an example an array of strings, representing names, is choosen.
A while ... do ... loop is used.
Data
const maxname = 1000;
var names : array[1..maxname] of string;
namecount : word;number of names in array
Function findname compares string s against the entries in array names.
If s is found, then the index of s is returned.
If no match is found, 0 is returned.
The function
function FindName(s : string) : word;
var i : word;
hit : boolean;
begin
hit := false;
i := 0;
while (hit = false) and (i < namecount) do
begin
inc(i);
hit := names[i] = s;
end;
result := i;
end;
The while..do.. loop repeats as long as no compare is found and the top of the array is not reached.
A convenient way of programming is also having the result of the function indicate if the search was successfull.
The index is returned as a var parameter.
Alternative search function
function FindName(var w : word; s : string) : boolean;
var i : word;
begin
i := 1;
while (i <= namecount) and (names[i] <> s) do inc(i);
result := i <= namecount;
if result then w := i;
end;
In this case, make sure that in Project Options/compiler "complete boolean evaluation" is set false.
So, if ( i <= namecount) is false, (names[i] <> s) comparison will not take place.
If no match is found, i will be incremented until it is greater then namecount.
A false result is returned.
|
|