|
 |
Delphi programming
filtering characters for names and passwords |
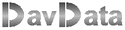 |
 |
 | download filter demo program |
 | download Delphi-7 project |
This article describes how to program character filters,
to allow only a selected set of characters to enter an Edit component.
Below is a form with two Edit boxes, one (nameEdit) for entering a name, a second (pwEdit) for entering passwords
Below the Edit components, the allowed characters are listed.
For the pwEdit, the property passwordChar is set to #149 during design time.
So each character entered here will be displayed as a big dot.
Both Edit components are placed on form1.
To be able to inspect a typed character, the keyPreview property of the form must be set true.
A typed character generates a TForm1.FormKeyPress(Sender: TObject; var Key: Char) event.
If the character key is not part of a predefined set, key is set to #0, character code 0, which is ignored.
Make sure the character #8 (backspace) is also allowed, else a backspace in the Edit box is not possisble.
Below is the complete Delphi-7 unit
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms,
Dialogs, StdCtrls;
type
TForm1 = class(TForm)
nameEdit: TEdit;
pwEdit: TEdit;
Label1: TLabel;
Label2: TLabel;
Label3: TLabel;
Label4: TLabel;
Label5: TLabel;
procedure FormKeyPress(Sender: TObject; var Key: Char);
private
{ Private declarations }
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
procedure namefilter(var ch : char);
begin
if not (ch in ['0'..'9','A'..'Z','a'..'z',#8]) then ch := #0;
end;
procedure pwfilter(var ch : char);
begin
if not (ch in ['!','#'..'&','*','-','/','0'..'9','A'..'Z','a'..'z',#8]) then
ch := #0;
end;
procedure TForm1.FormKeyPress(Sender: TObject; var Key: Char);
begin
if activecontrol = nameEdit then namefilter(key);
if activecontrol = pwEdit then pwfilter(key);
end;
end.
|
|