|
 |
character string encryption |
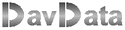 |
 |
 | download encryption exerciser program |
 | download complete Delphi-7 project |
Introduction
Sometimes is it desirable to save text in an unreadable form, as is the case with passwords.
This article describes a simple way to encrypt a character string.
The Method
A character string is regarded as groups of 4 characters.
So, characters 1..4 make group 0, characters 5..9 make group 1, and so forth.
Each group of 4 characters is packed into a 32 bit integer (DWORD).
Then the bits of this DWORD are interchanged.
Next the DWORD is regarded as groups of 6 bits.
Groups 0..4 are 6 bits wide, group 5 has 2 bits left.
A 6 bits group is an index into a preset string of 64 characters, so each 6 bit value
yields a character. These characters together make the encrypted string.
The advantage of this method is , that a character has no fixed translation,
because it depends on the neighbouring characters in the string.
Note, that 4 characters (each 8 bits in size) translate to 6 characters.
figure below shows the data flows
Detailed description
We want to encrypt the string 'hello there'
The first 4 characters are placed in variable dd.
Variable i steps through the 32 bit DWORDs, j counts 0..3 for the 4 characters in a DWORD.
When DWORD dd has it's 4 characters then variable k steps through all 32 bits of dd and loads bits
11, 22, 1, 12, 23, 2, 13, ..... {counting in increments of 11, modulo 32} and places these bits in
positions 0, 1, 2, 3, ... of DWORD ee.
ee is now regarded as fields of 6 bits.
Then k steps from 0..5 to extract the 6 bits fields.
Variable n is the index into string encstring.
encstring[n] provides the final character to be placed in the result string.
encstring[ ] is initialized at the start of the project.
it is preset to '. / 0123456789ABCD....XYZabcd...xyz' in total 64 characters.
For decoding an encrypted string, the opposite happens.
Please refer to the source code for details.
Exerciser program
A Delphi-7 project has been build to exercise the encoding / decoding of strings.
A string may be entered in the source text box.
When the encode button is pressed, this text is shown in the dest statictext in the encrypted form.
"X buttons allow for clearing the text boxes.
Clear the original source code, then press decode to show the source text again.
In the project, a separate unit encrypt_unit holds the code for the encryption / decoding of a string.
Below is a snapshot of the exerciser program at work.
|
|