|
 |
Searching solutions for m*[ABC...]=[...CBA] |
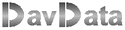 |
 |
download program
download Delphi project
Recently I came across this problem:
Find decimal digits A,B,C,D such that: 4[ABCD]=[DCBA]
A solution is 2178 because 4*2178 = 8712.
Question arised: are there more examples of this property?.
So, investigate numbers for m*[ABCD...] = [...DCBA]
This program investigates.
The number of digits [ABCD..] varies from 2..8.
The multiplier m range is 2..11.
Data
const digvalue : array[1..8] of dword = (1,10,100,1000,10000,100000,1000000,10000000);
Nmax : array[1..8] of dword = (9,98,987,9876,98765,987654,9876543,98765432);
Nstart : array[1..8] of dword = (0,01,012,0123,01234,012345,0123456,01234567);
type TABCD = array[0..8] of byte;
var ABCD : TABCD;
digcount : byte;
busy : boolean;
XF : boolean; //exit flag
The digvalue constants are needed to make an integer value from the digits.
Nmax is the largest number to be investigated for a certain digitcount.
Nstart is the first number.
ABCD array holds the digits of the number
digcount is the number of digits.
busy is true as long as numbers are tested.
Purpose is to prevent a new search while the process is not yet finished.
XF (exitflag) stops the search.
The operation needs some simple procedures and functions
which are described below:
Unpack
Takes the digits out of the number and place them in byte array ABCD[ ]
procedure unpack(w : dword);
//w --> ABCD
var i : byte;
begin
for i := 0 to digcount-1 do
begin
ABCD[i] := w mod 10;
w := w div 10;
end;
end;
Extracts the digits in w and put them in array ABCD.
PackReverse
Make a number from reversed digits of array ABCD[ ].
function packReverse : dword;
//ABCD --> DCBA --> word
var d : dword;
i : byte;
begin
d := digvalue[digcount];
result := 0;
for i := 0 to digcount-1 do
begin
result := result + d*ABCD[i];
d := d div 10;
end;
end;
The digits in array ABCD[ ] must be tested for uniqueness.
This is done by
function unique : boolean;
//test digits of ABCD[ ] are unique
var dflag : array[0..9] of boolean;
a,i : byte;
begin
result := true;
for i := 0 to 9 do dflag[i] := false;
i := 0;
while result and (i < digcount) do
begin
a := ABCD[i];
if dflag[a] then result := false
else begin
dflag[a] := true;
inc(i);
end;
end;
end;
Example: if digit '9' occurs, dflag[9] is set.
If digit '9' occurs again dflag[9] is found true and the function exits false.
Testing a number
function testnumber(n : dword) : byte;
//test n for solution
//result = 0 : no solution
//result > 0 : multiplier
var m : byte;
N2 : dword;
begin
result := 0;
N2 := packreverse;
m := 2;
repeat
if (m*n) = N2 then
begin
result := m;
exit;
end;
inc(m);
until m = 12;
end;
Searching all numbers
procedure search;
var i,mptest : byte;
number : dword;
timer : dword; //proc messages
begin
mptest := 0;
for i := 2 to 8 do
begin
timer := 0;
digcount := i;
showparams(i);
number := Nstart[i];
repeat
unpack(number);
if unique then
begin
mptest := testnumber(number);
if mptest > 0 then reportsolution(number,mptest);
end;
inc(number);
if timer = 0 then
begin
application.ProcessMessages;
timer := 10000;
end
else dec(timer);
until (number > Nmax[i]) or XF;
end;//for i
end;
Control
The search is started by a button click.
procedure TForm1.Button1Click(Sender: TObject);
//GO button
begin
if busy then exit;
//
XF := false;
busy := true;
memo1.clear;
search;
busy := false;
msglabel.caption := 'end';
end;
Reset and interrupting a search
procedure reset;
begin
xf := true;
form1.Memo1.clear;
form1.msglabel.caption := 'start';
end;
procedure TForm1.Button2Click(Sender: TObject);
//reset button
begin
reset;
end;
The timer variable in procedure search triggers Windows message processing
from time to time, so pressing te RESET button during a search is recognized.
These are the central procedures and functions.
For all procedures and details please refer to the Delphi project.
|
|